Javascript part 8
Today we talk about switch statements and Arrays.
Switch statements are used for basic comparison or controlled structure; instead of a long “if statement,” I would do a switch statement if I was you. Basically, makes it easier for when you have different actions for each different condition.
let me show you an example:
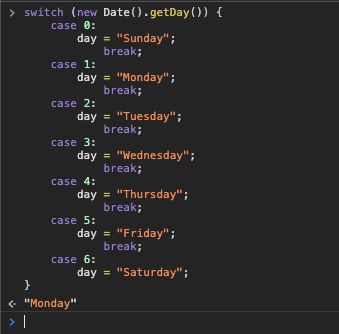
ok, first we always start it with a “switch ” keyword, then parenthesis to compare it against something. (All we are doing is asking what day is it)
“getDay()” returns a number for the day of the week(this is a javascript method already made inside javascript).
We set those numbers with a “case,” number. if the function “getDay()” returns any of these numbers(which will return a number, not an actual day) we simply output the day of the week.
We also put “breaks” into it because if you didn't it would skip over all the information landing on the last day before the break. the example below; if you miss the “break.” it would continue until it sees that break then return the value.(it's Monday today, but returns Tuesday since we don't have breaks)

Another example below, I set a variable to “animal” and the value to “giraffe.” Then used that variable to check in my Switch statement.
We also see that the “break” isn't shown till after the “console.log.” That's because we want it like this, to show in the console like a list.
It's like saying if you see a “Cow, Giraffe, Dog, Pig,” put it in this category, then be done with the switch statement. (It will return “this animal will go on noahs ark.” since the animal is “Giraffe”)
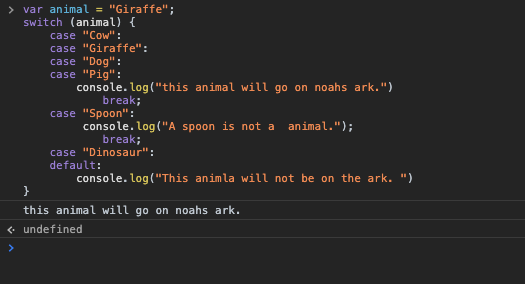
Also, we see something different here; we see “DEFAULT” at the very bottom. this is a good habit to pick up, catches the “case statement” almost like a safety net, so you don't get errors.

In the example above, I changed what animal value was. I changed it to “Piggy,” so then it looks through everything and doesn't see any cases with that name.
so default to the rescue it will console.log “This animal will not be on the ark.”
Arrays:
Arrays can hold multiple pieces of data in one place. Arrays are like boxes you keep things in and label.
There are different methods to get the “things” out of those boxes.
We create an array with “[]” square brackets and a “,” comma between each element or data input(look below). You can put strings, numbers, objects, booleans, any type.
Side note: we can also put arrays inside arrays.

one of the common ways to access these arrays is through indexes. Indexes are where certain elements sit in the array(reminder arrays start with 0, not 1. so if we wanted pig from the example below we would of had to put 0).

See above; we said animal[1](most people would think “pig” would have been chosen, but “pig” is 0).
“pig” = 0 index
“lama” = 1 index
“dog” = 2 index
Moving on, we can also change elements with indexes. In the example below, we grab “dog,” which is the element 2 and we replace it with “cat.” Then when we call the “animal” variable, you now see that “cat” has taken the “dog” place in position 2.
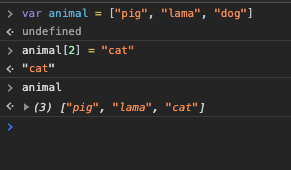
More intense array:
Now we get into a nested array situation in the below example.
We set the “house” variable to two arrays inside one array.
“mid century,” and the year 1950 is one array.
“bohemian look,” and the year 2020 is the second array.

To get these arrays out, we could simply call house[1], check below what we get.

We got one array but not the information inside that array. So we would have to put two indexes to get the data out; check below.

Now we were able to get “bohemian look” out by sayin: which array do we want, mid-century or bohemian?
We want the bohemian out, so we use index 1. Then we say, ok, what do we want out of the bohemian array? just the name… ? We then put the 0 indexes to get that specific data out.”
Next up, we tackle the way you would change a deeply nested array value.

very simple actually if we look above, we do it just like we did it before. we call it with house[1] gets second array, then inside that aray i want the first item house[1][0]. Then simply chnage the value like so:
house [1][0] = to “BOHEMIAN SHEEK”
It will now be set in that exact index… FOREVER!
One of the methods that all the arrays have is a “forEach” method.
This method will cycle each element of the array, run a function on it and output those elements. look at the example below

All we did was output or console.log each element in the array.
Saying “ take num variable and use this function forEach on each element.” the “x” you can call anything you want just any name, for each element in the array. Then it runs the function on each element in the array.
OK thank you for that bite size fun, dont forget to check out other articles.
check out some javascript….
Javascript 1 (variables and data types)
Javascript 2 (numbers and strings)
Javascript 3 (bracket notation and 20 diff string methods)
Javascript 4 (functions and how they work)
Javascript 5 (hoisting, comparison operators, and if-else statements)
Javascript 6 (diff. Equal signs, null, and undefined)
Javascript 7 (logical operator, &&, || and ternary operators)
Javascript 8 (switch statements and arrays)
Javascript 9 (commonly used arrays in javascript)
Javascript 10 (Math. And parseInt Usage)
Javascript 11 (for loops and nested for loops)
Javascript 12(while loops and for..in and for..of loops)
Javascript 13(8 diff array methods)
Javascript 14(objects and ways to use objects)
Javascript 15(JSON and fetch request)
Javascript 17(strict mode and error handling)
Javascript 18(setInterval/setTimeout and Dates)
React info…
React part 1 (How to start a react app)
React part 2 (components and dynamic values)
React part 3 (Multiple components and small styling)
React part 4 (click events with functions and react dev tools)
React part 5 (link to: useState hook and how to output lists in react)
React part 6 (Props and reusable components)
React part 7 (passing functions as props and use effect hook)
React part 9 (Fetching data with JSON)
React part 10 (loading bar and fetch errors)
React part 12(React Router Dom, Exact, and Links)
The Social Media…